Learn Python Coding Language - The Gateway to Programming Excellence
Discover why Python is the most popular and versatile programming language today.
Get StartedWhy Choose Python?
Python is a versatile programming language used in web development, data science, machine learning, and more. Its simplicity, extensive libraries, and strong community support make it an excellent choice for beginners and professionals alike.
Key Features of Python
Easy to Learn
Open-Source
Extensive Libraries
Cross-Platform
Simple Syntax
Python's syntax is clear and intuitive, making it easy to read and write code.
print("Hello, World!")
Extensive Libraries
Python has a vast ecosystem of libraries for various purposes, from web development to data analysis.
import numpy as np
array = np.array([1, 2, 3])
Object-Oriented
Python supports object-oriented programming, allowing for modular and reusable code.
class Dog:
def bark(self):
print("Woof!")
Dynamic Typing
Variables in Python can hold different types of data without explicit declaration.
x = 5
x = "Now I'm a string"
Interpreted Language
Python code is executed line by line, making debugging easier.
# No compilation needed
# Just run your .py files
Cross-platform
Python runs on various operating systems, including Windows, macOS, and Linux.
# Write once, run anywhere
# (with Python installed)
Well-Known Python Libraries and Their Use Cases
NumPy
Fundamental package for scientific computing with Python.
Use Cases:
- Multidimensional arrays
- Linear algebra
- Fourier transforms
Pandas
Data manipulation and analysis library.
Use Cases:
- Data cleaning
- Time series analysis
- Data visualization
Matplotlib
Comprehensive library for creating static, animated, and interactive visualizations.
Use Cases:
- Line plots
- Scatter plots
- Histograms
Scikit-learn
Machine learning library for classical ML algorithms.
Use Cases:
- Classification
- Regression
- Clustering
TensorFlow
Open-source library for machine learning and artificial intelligence.
Use Cases:
- Deep learning
- Neural networks
- Computer vision
Django
High-level Python web framework that encourages rapid development and clean, pragmatic design.
Use Cases:
- Web applications
- Content management systems
- RESTful APIs
Flask
Lightweight WSGI web application framework.
Use Cases:
- Microservices
- RESTful APIs
- Small to medium web applications
Requests
Elegant and simple HTTP library for Python.
Use Cases:
- API integration
- Web scraping
- HTTP requests
Pillow
Fork of PIL (Python Imaging Library) with easy-to-use image processing capabilities.
Use Cases:
- Image resizing
- Format conversion
- Drawing
About Python
History and Evolution
Python was created by Guido van Rossum and first released in 1991. It was designed with an emphasis on code readability, and its syntax allows programmers to express concepts in fewer lines of code than would be possible in languages such as C++ or Java.
Over the years, Python has evolved significantly, with major versions including Python 2 and Python 3. The language continues to grow in popularity due to its simplicity, versatility, and the strength of its community.
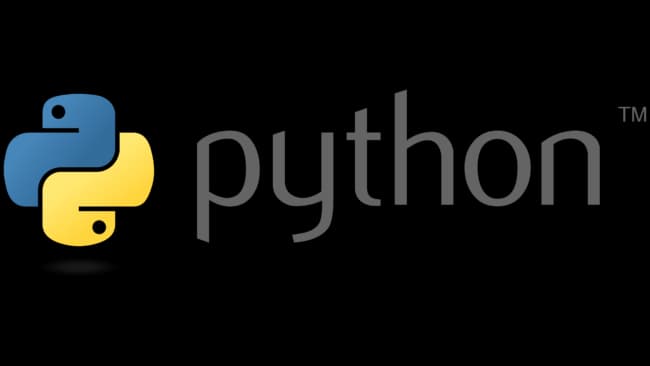
Why Python is Great for Beginners and Professionals
- Easy to learn and read, with a clean and straightforward syntax
- Extensive standard library and third-party packages for various tasks
- Strong community support and comprehensive documentation
- Cross-platform compatibility (Windows, macOS, Linux)
- Versatility in application (web development, data science, AI, automation, etc.)
Python Coding Language use cases
E-commerce website
Build an online store with Django or Flask
Data analysis dashboard
Use Pandas and Matplotlib to visualize business insights
Spam email classifier
Train a model to detect spam messages
Web scraping tool
Extract data from websites for research or automation
2D arcade game
Create a simple platformer with Pygame
Cryptocurrency trading bot
Automate trading strategies on crypto exchanges
AI-powered text generation
Use GPT models to create blog posts, stories, or product descriptions
Real-time chat application
Implement WebSockets for live messaging
Customer segmentation
Analyze shopping behavior for targeted marketing
Fraud detection system
Identify fraudulent transactions using AI
Automated report generator
Compile and email business reports automatically
AI-powered chess game
Implement a chess engine with Minimax or reinforcement learning
Portfolio risk analysis
Calculate risk and return metrics for investments
AI chatbot for customer service
Generate natural responses to customer inquiries
RESTful API for mobile apps
Develop a backend API to serve mobile applications
Social media trend analysis
Extract insights from Twitter or Instagram data
Image recognition for security
Use computer vision to detect faces or objects
CI/CD pipeline automation
Automate software testing and deployment
Multiplayer online game
Build a real-time game server using Python
Stock price prediction
Use machine learning to forecast market trends
AI-generated art and images
Create artwork using models like Stable Diffusion
Content management system (CMS)
Create a custom CMS for blogs or company websites.
URL Shortener
Create a service like Bit.ly to shorten long URLs.
Medical data analysis
Process healthcare records to detect disease patterns.
Real-time stock market analytics
Visualize stock movements with dynamic graph.
Chatbot for customer support
Implement a conversational AI assistant.
Personalized recommendation engine
Suggest movies, books, or products.
File organizer
Automatically sort and rename files based on rules.
Auto-login bot
Fill forms and log in to websites without manual input.
Educational game for kids
Develop a math-based learning game.
Automated budgeting app
Track expenses and suggest saving strategies.
Tax calculation tool
Automate tax estimations based on income and deductions.
Python coding language Tutorials
Getting Started with Python
Hello, World! in Python
Here's a simple example to get you started with Python:
print("Hello, World!")
# Output:
# Hello, World!
This simple program demonstrates how easy it is to print text in Python.
Beginner Tutorials
Video Tutorials
Ready to Practice?
Start coding and improve your Python skills with interactive exercises.
Practice on HackerRankF.A.Q.
Everything you need to know about Python Coding Language
What is Python coding language?
+Python is a high-level, interpreted programming language known for its simplicity and readability. It supports multiple programming paradigms, including procedural, object-oriented, and functional programming.
Is Python free to use?
+Yes, Python is open-source and free to use, even for commercial purposes. You can download and install Python from the official website (python.org) at no cost.
What can I build with Python?
+Python is versatile and can be used for various applications, including web development, data analysis, artificial intelligence, machine learning, scientific computing, automation, game development, and more.
Is Python good for beginners?
+Yes, Python is an excellent language for beginners. Its simple syntax, readability, and extensive documentation make it easier to learn compared to many other programming languages.
Where can I learn Python?
+There are many resources to learn Python, including online tutorials, interactive platforms, books, and video courses. Some popular options include the official Python documentation, Codecademy, Coursera, edX, and various YouTube channels dedicated to Python programming.
What are some popular Python frameworks?
+Some popular Python frameworks include Django and Flask for web development, NumPy and Pandas for data analysis, TensorFlow and PyTorch for machine learning, and Pygame for game development.
How does Python compare to other programming languages?
+Python is known for its simplicity and readability. It often requires fewer lines of code compared to languages like Java or C++. Python is interpreted, which can make it slower for certain tasks, but it's highly productive for development. Its extensive library ecosystem is a significant advantage.
Can I use Python for web development?
+Yes, Python is widely used for web development. Frameworks like Django and Flask make it easy to build web applications. Python can be used for both backend development and full-stack development when combined with frontend technologies.